Drag and Drop Interactions
Everyday workflows often require not only the usage of VidiEditor alone but combine a chain of steps over different frontends. An easy example is the usage of MediaPortal optimized for searching and collection media for further tasks. This for example could be editing in VidiEditor the collected media in MediaPortal. Obviously its more convenient if a user can drag media from the one application to the other using to browser tabs then searching the media again or creating dedicated collections. Therfore VidiEditor allows one to use drag and drop interactions allowing to import media into different parts of the VidiEditor frontend for further usage.
The integrating application must simply implement a drag an drop interaction and hand over a payload in the defined data structure.
Possible Use Cases
VidiEditor accepts the following data based on the VidiCore data model typically containing the media data:
Item
Single Item
Partial Item
Multiple Items
Multiple partial items
Collections
Single Collection
Multiple Collection
VidiEditor Project Collection
Interface
The basic interface used for drag and drop interaction looks as follows:
export interface ItemData {
itemId: string,
tcIn?: string, // { number of samples }[@{ textual representation of time base }] 50@PAL, 50@NTSC, 50@25:1
tcOut?: string, // { number of samples }[@{ textual representation of time base }] 50@PAL, 50@NTSC, 50@25:1
}
export interface DropData {
externalData: {
item?: ItemData[], // list of item id and partial start and end time if provided
collection?: string[], // collection id
veProject?: string, // VE project collection id
}
}
Drop Zones
VidiEditor provides 3 possible drop zones for different cases, especially designed to easily hand over media from an application into VidiEditor for further editing. The available drop zones are:
Management Area - Allows to enrich items or collections to the project bin
Source Media Player - Allows to preview single items in the source player directly
Timeline - Media dropped directly onto the timeline area will be added to the current timeline, if the item does not exist in bin, then will add into bin as well. Media will be added at last frame + 1 of currently opened timeline
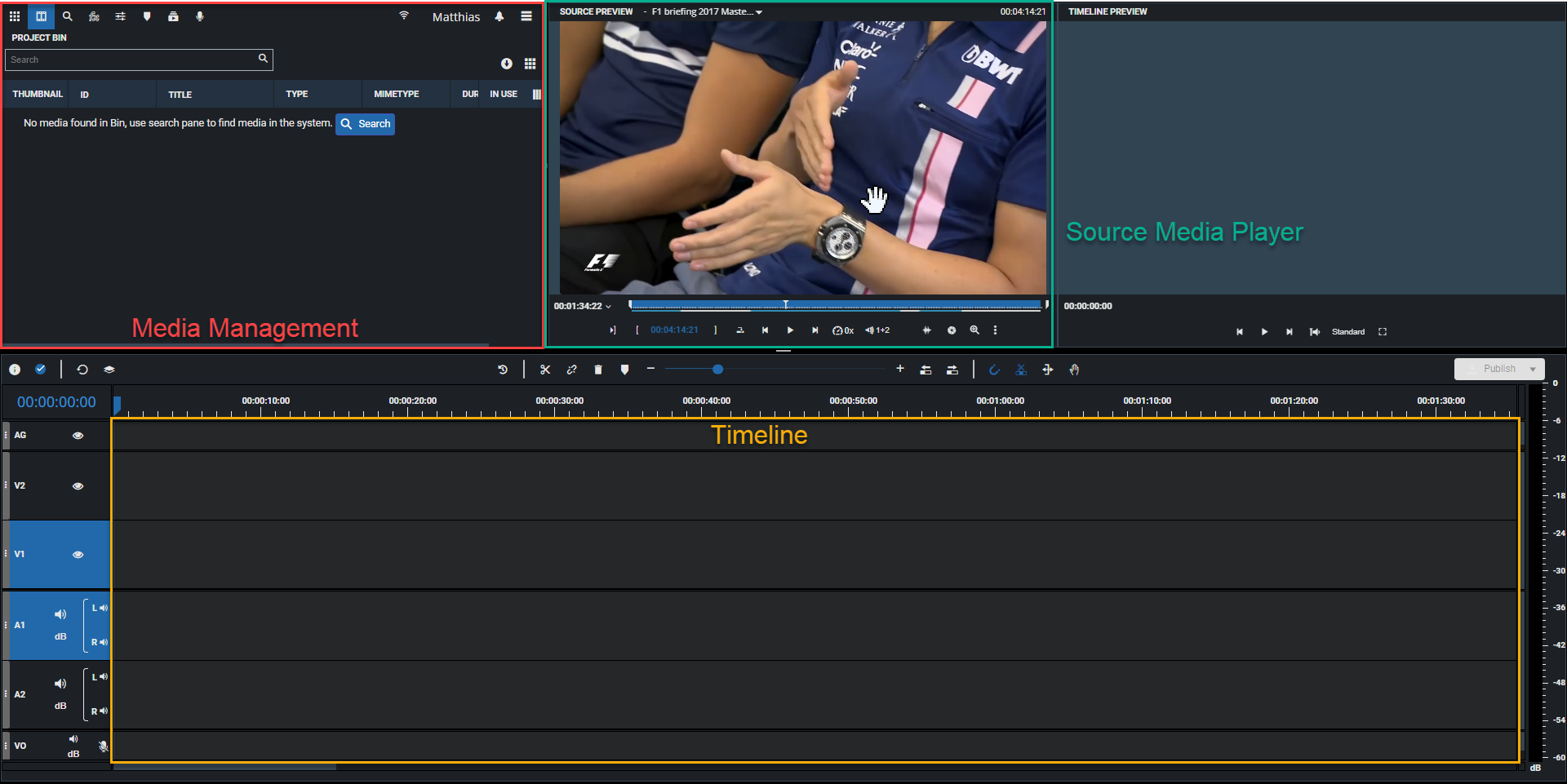
Please note that not every media makes sense on every drop zone. For example playback of a collection in source player will not work out of the box as playback as the data would need to be massaged beforehand - the first item of the collection would be load instead. Or as another example dragging a VidiEditor project onto a timeline will not combine it to the opened timeline but would ask the user to switch the project.
Data Structure
The drag and drop interaction must hand over the correct data to the drop zone being able to act on it. When initiating a drag event from an application, ensure that the DataTransfer
object is populated with the appropriate format (‘text/plain’). A sample can look as follows based on above mentioned interface:
const data = {
externalData: {
item: [
{
itemId: "ITEM-VX-462769" // full duration
},
{
itemId: "ITEM-VX-236876", // partial duration
tcIn: "50@PAL",
tcOut: "100@PAL",
},
],
// collection: [
// "COLLECTION-VX-451618",
// "COLLECTION-VX-451620",
// ],
// veProject: "COLLECTION-VX-5451"
}
};
event.dataTransfer.setData('text/plain', JSON.stringify(data));
HTML example
To ease up implementations a small HTML example should be given illustrating the basic usage for VidiEditor drag an drop support:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Drag Source</title>
<style>
#draggable {
width: 100px;
height: 100px;
background-color: lightblue;
cursor: grab;
}
</style>
</head>
<body>
<div id="draggable" draggable="true">Drag me</div>
<script>
document.addEventListener('DOMContentLoaded', (event) => {
const draggable = document.getElementById('draggable');
draggable.addEventListener('dragstart', (event) => {
const data = {
externalData: {
item: [
{
itemId: "ITEM-VX-462769"
},
{
itemId: "ITEM-VX-236876",
tcIn: "0@PAL",
tcOut: "50@PAL",
},
],
// collection: [
// "COLLECTION-VX-451618",
// ],
// veProject: "COLLECTION-VX-5451"
}
};
event.dataTransfer.setData('text/plain', JSON.stringify(data));
});
});
</script>
</body>
</html>
Flow Limitations
It should be mentioned that some limitations exist in the flow that is essentially designed to easily get media from one application into VidiEditor easily.
Landing Page Vs Open Project
When no project is open in VidiEditor, only the management area is enabled as a drop zone. If an item is dropped, VidiEditor will prompt the user to either create a new project or add the item/collection to an existing project, based on user selection.
Not supported media
If media is dropped to VidiEditor that is not handable in general VidiEditor will display an error message. Typical examples are:
Dropped media ha no configured proxy shape for VidiEditor usage
Not supported frame rate or media format or media has different frame rate the open project
Alpha channel expected but not supported on Proxy video
Collections handed over of not supported type such as a Placeholder collection